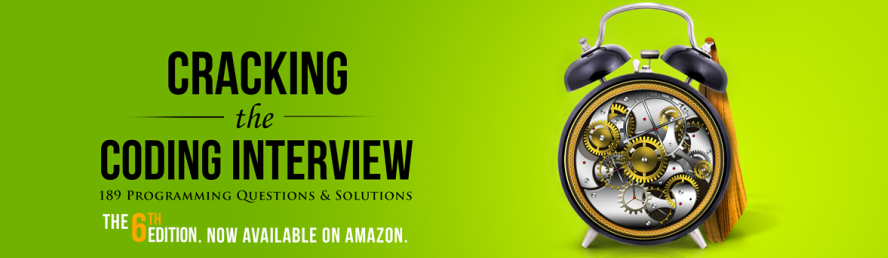
Problem
A left rotation operation on an array shifts each of the array’s elements 1 unit to the left. For example, if 2 left rotations are performed on an array [1,2,3,4,5]
, then the array would become [3,4,5,1,2]
. Note that the lowest index item move to the highest index in a rotation. This is called circular array.
Given an array a of n integers and a number, d perform d left rotations on the array. Return the updated array to be printer as a single line of space-separated integers.
Function Description
Complete the function rotLeft in the editor below.
rotLeft has the following parameter(s):
- int a[n]: the array to rotate
- int d: the number of rotations
Returns
- int a'[n]: the rotated array
Input Format
- The first line contains two space-separated integers n and d, the size of a and the number of left rotations.
- The second line contains n space-separated integers, each an a[i]
Constraints
1 <= n <= 105
1 <= d <= n
1 <= a[i] <=106
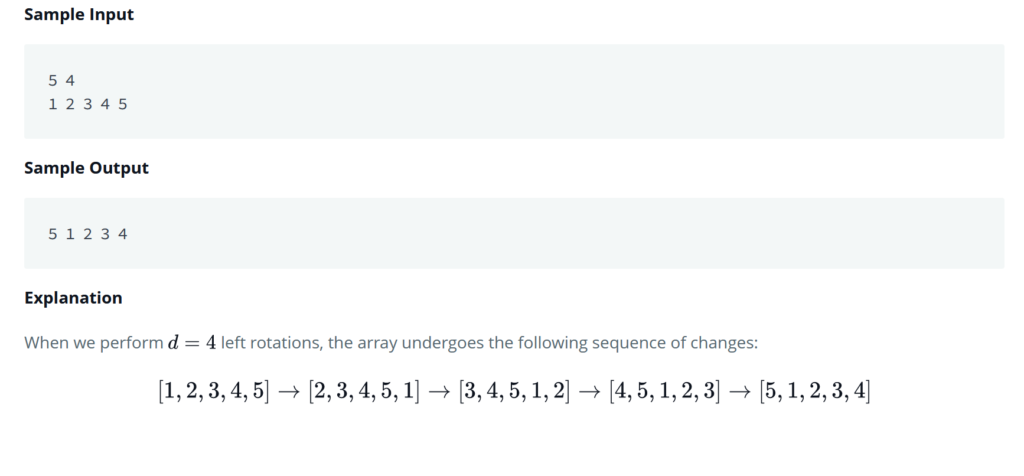
Solution
package org.span; import java.io.IOException; public class ArrayLeftRotation { static int[] rotLeft(int[] a, int d) { for (int i = 0; i < d; i++) { int t = a[0]; System.arraycopy(a, 1, a, 0, a.length - 1); a[a.length - 1] = t; } return a; } public static void main(String[] args) throws IOException { int d = 4; int[] a = {1, 2, 3, 4, 5}; int[] result = rotLeft(a, d); print(result); } public static void print(int[] a) { for (int i = 0; i < a.length; i++) { if (i > 0) System.out.print(" " + a[i]); else System.out.print(a[i]); } } }